Technical Blog - JavaScript Fundamentals
What are functions in JavaScript
It is a set of statements that performs a task and is the main building block of a JavaScript file.
Functions are defined by the following:
- The name of the function. eg:
function nameOfTask ()
- The list of parameters to the function (if any, can be empty as well), enclosed in a parantheses and separated by commas. eg.
function nameOf (param1, param2)
- The statements that define the function, enclosed in curly brackets:
function myFunction (param1, param2) { /* Code goes in here */ }
HTML (Hyper Text Markup Language)
It is the coding language used for
Relationship with JavaScript: everytime a user performs an actvity on the webpage, actions occur (eg.scrolling, clicking)
To make web-pages interactable to the user, we create different types of
In order to create events, we must code
CSS (Cascading Style Sheet)
What is it? It is the coding language used that applies presentation (styling, layout, animation) to the web-page.
Relationship with JavaScript: we can create programmes in the form of scripts that instructs the web-page to change its style drammatically.
We can code functions within the programme, that targets certain elements on the HTML file and have them add a new attribute.
Control Flow and Loops
Control Flow
It is the order in which the computer executes the statements in a script of code. Code normally runs in order from first to the last line unless there are conditionals and loops.
Real Life Example: we are given a recipe to make beef empanadas, so follow the instructions set out in the order they are written. This is similar to a script of code, the computer will follow the order it is written.
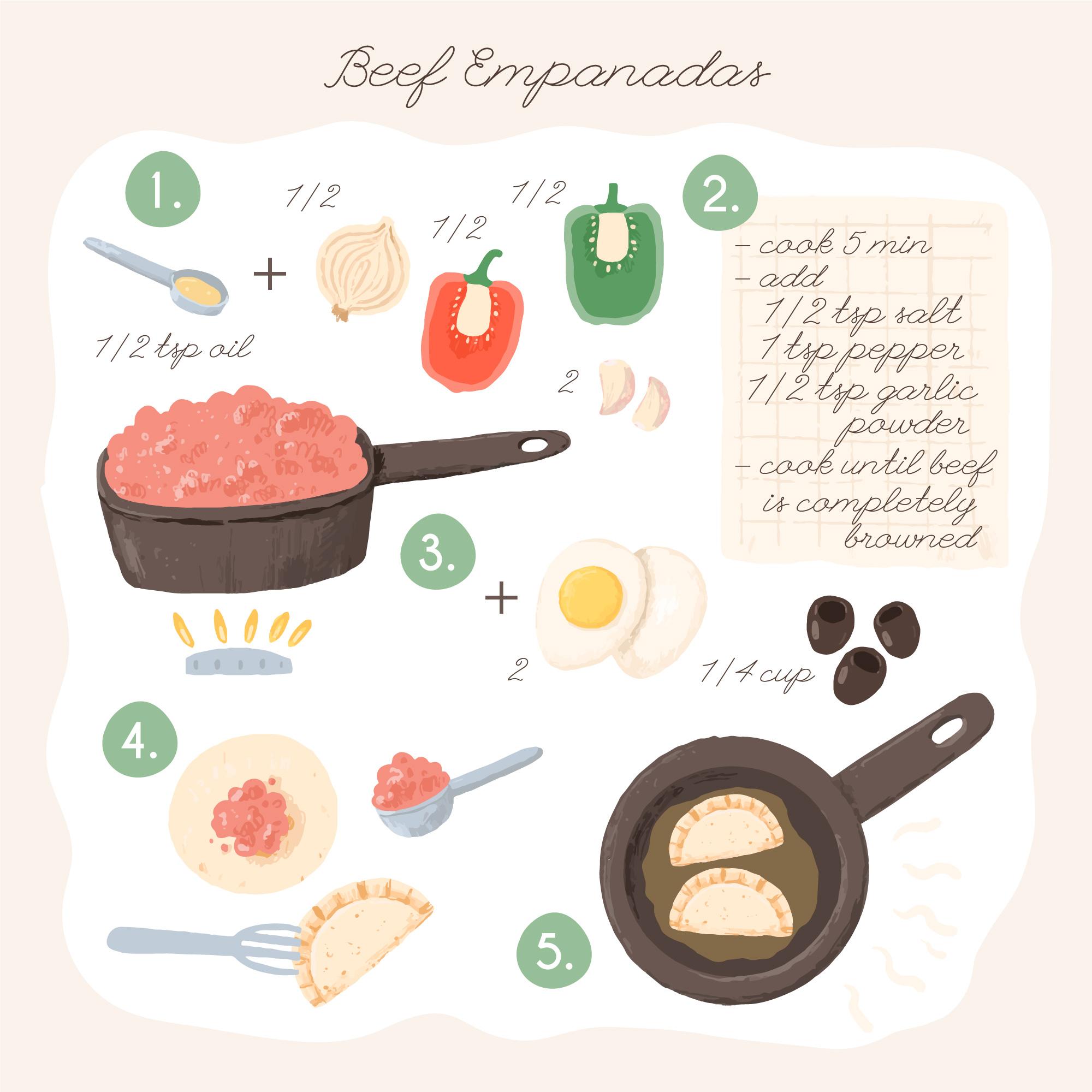
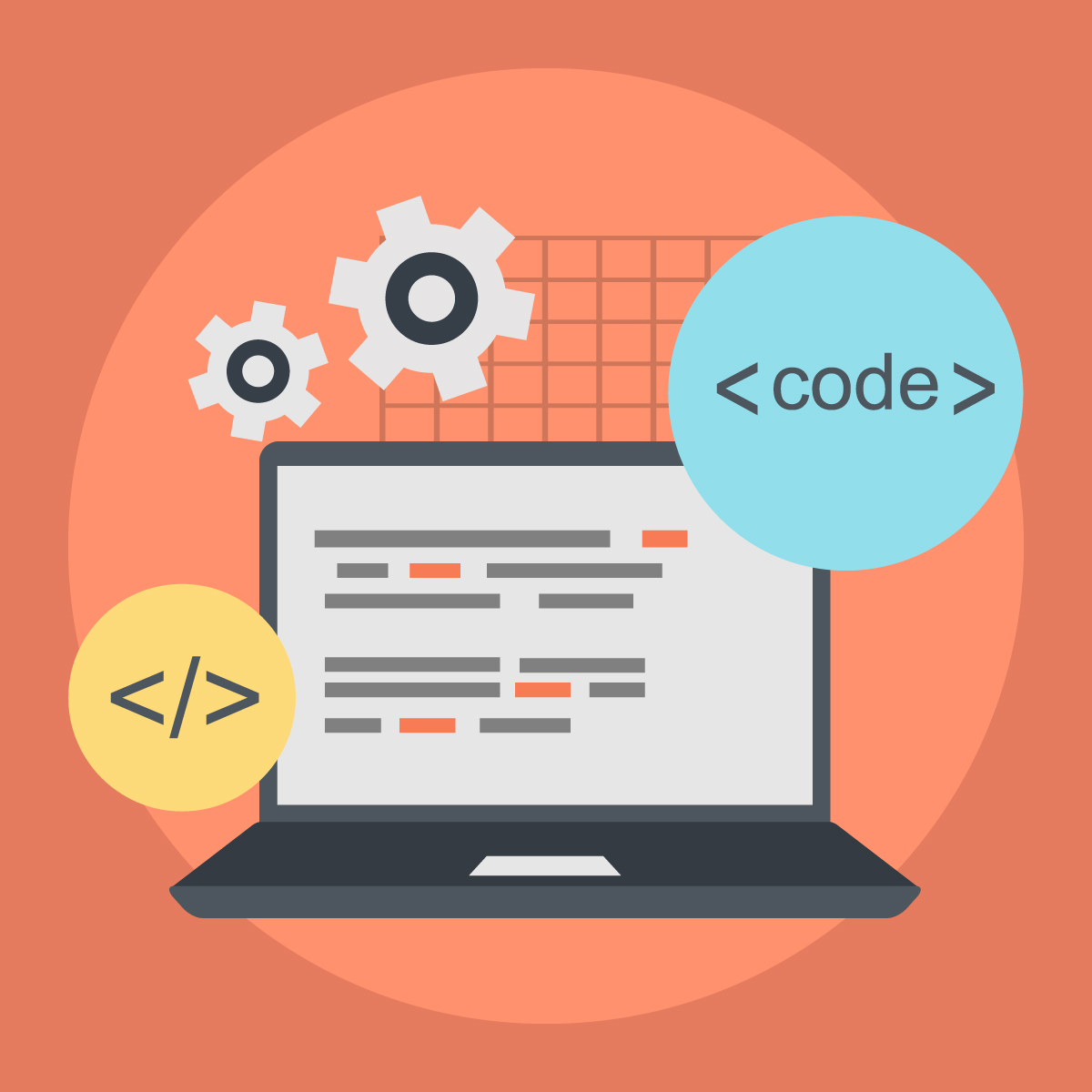
Coding Example: When we write code on an editor such as Visual Studio Code, it is normally numbered. Within the computer, is a compiler that goes through each line and then translate late it into the output that's either seen on the console log or on the web page itself. In a way, we are the programmers instructing the computer to do something.
Loops
A sequence of instructions that is continually repeated until a certain condition is reached. These instructions normally involve finding an item within a set of data and the conditions involved having a counter reach a certain number of repetitions.
Coding Example: most of the applications of loops involves storing a set of data in an array and then filtering them via a loop. Inside a loop are nested conditionals used to filter the desired data necessary.
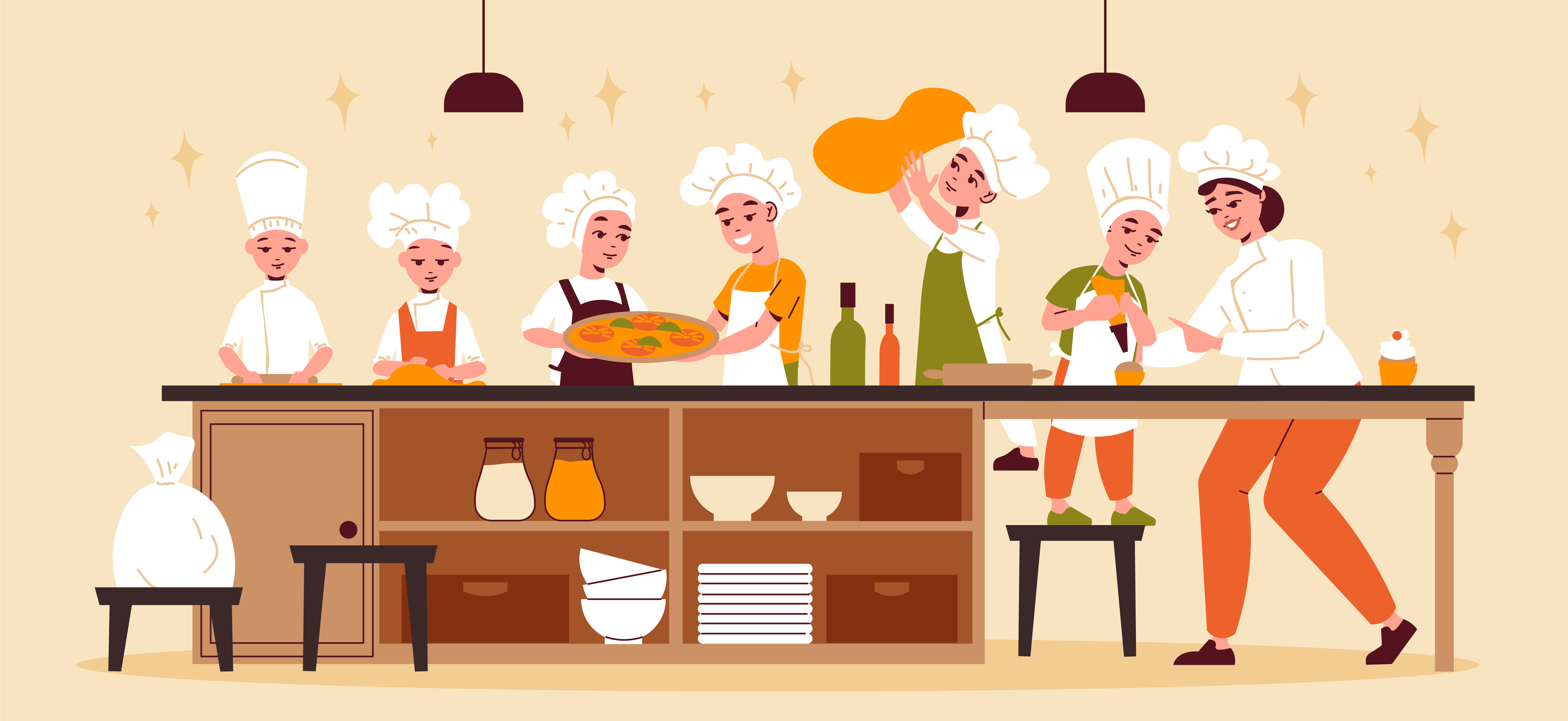
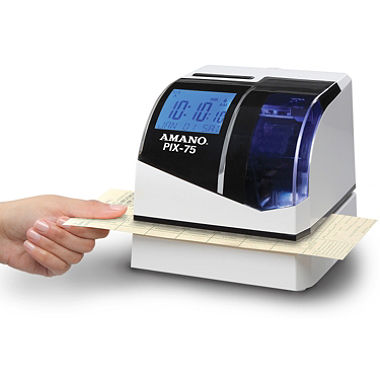
Real Life Example: we can use a real-life person who works at place, five days a week and 8 hours a day. For example, a baker works at a local bakery everyday, making bread and has only 8 hours to do it. The type of bread he makes depends on the demand of customers and his manager, which is set out similar to conditional statements inside the loop. The baker has a timestamp of his daily shift. In a way, the baker's working days are like loops, which could end after 8 hours or depending on whether he completes all of his tasks much like conditional statements.
Acessing Data from Arrays and Objects
Objects
Definition: in Objects, data is stored in a key-value pair sequence.
For example, name : 'Benedict'
- name is the key and 'Benedict' is the value. They can be accessed in any order.
If we want to acessed each object, then we can either use the dot operater(.)
followed by the key name obj.name
or using a square brack along with a key in string format obj['name']
to accessed
the value.
Arrays
In Array, data is stored in an ordered sequenence and data can be accessed using a numerical index.
An example of an array would be const array = [1 , 2, 3, 4, 5]
. To access the number 1, we must type console.log(arr[0])
.
The first element is stored in index 0 while the last element of 5 is at index 4.
DOM (Document Object Model)
Definition: It is language interface (in the form of abbreviated code called API) used to access the elements contained on the HTML document. It can be used to connect the HTML document with the JavaScript language.
Usage: We can use DOMs in a similar way that we would access an object, through acessing the dot operator(.)
In JavaScript, we can write a function that contains a tag that scans the content of a web-page. This code involves using a DOM to achieve that. We can make this function interactable by adding a button component to the page and initiating this function once a user presses it.
For example, we can change the text colour of this paragraph, to give it a green colour by writing a CSS code for green-text, then a JavaScript code with function for toggling to green and back to black
that contains the DOM API code for document.getElementById
and classList.toggle()
and then adding this into the button component as a click event.